Introduction
Python programming opens the door to a world of infinite prospects, and one basic job that usually stands earlier than us is extracting distinctive values from a listing. Getting distinctive values from a listing is a standard job in Python programming. Identical to every line of code has its distinctive goal, so do the weather in a listing, and discerning the singular gems from the muddle of duplicates turns into an important ability.
Distinctive values check with components in a listing that happen solely as soon as, with out duplicates. On this article, you’ll study A-Z about varied strategies for acquiring distinctive values from a listing and focus on their significance in numerous situations.
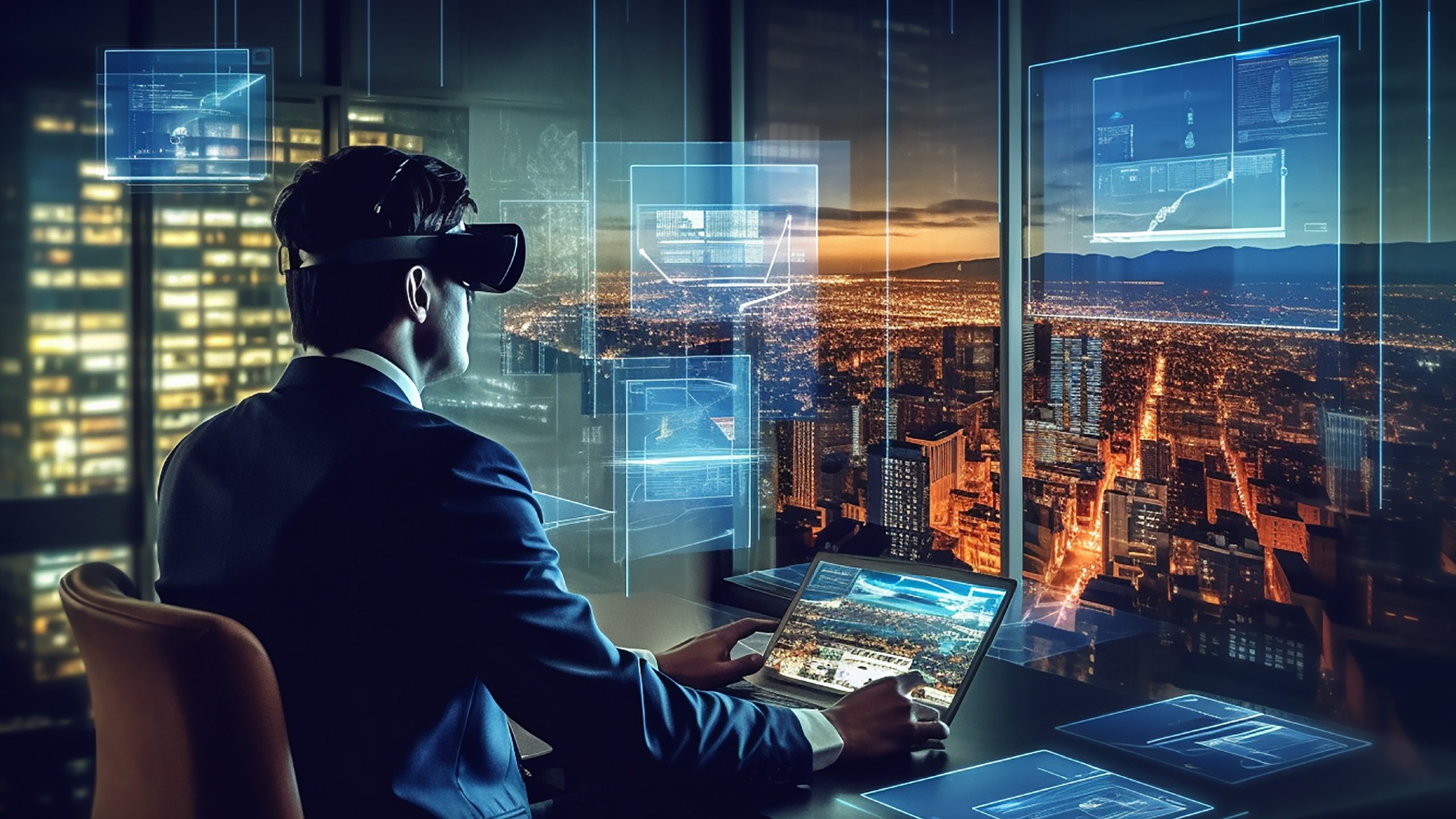
Why is Getting Distinctive Values Essential?
Acquiring distinctive values from a listing is essential in lots of programming duties. It permits us to remove duplicate entries, simplify knowledge evaluation, and enhance the effectivity of our code. Whether or not working with massive datasets, performing statistical evaluation, or manipulating knowledge buildings, having distinctive values can present correct and significant outcomes.
Strategies to Get Distinctive Values from a Record Utilizing Python
Utilizing the set() Operate
Python’s set() operate is a robust instrument to acquire distinctive values from a listing. It mechanically removes duplicates and returns a set object containing solely the distinctive components. We are able to then convert this set again into a listing if wanted.
Instance
my_list = [1, 2, 3, 3, 4, 5, 5, 6]
unique_values = listing(set(my_list))
print(unique_values)
Output
[1, 2, 3, 4, 5, 6]
Utilizing Record Comprehension
Record comprehension is one other concise and environment friendly technique to get distinctive values from a listing. We are able to filter out duplicates and procure solely the distinctive values by iterating over the listing and checking if a component is already current in a brand new listing.
Instance
my_list = [1, 2, 3, 3, 4, 5, 5, 6]
unique_values = [x for i, x in enumerate(my_list) if x not in my_list[:i]]
print(unique_values)
Output
[1, 2, 3, 4, 5, 6]
Utilizing the dict.fromkeys() Methodology
The dict.fromkeys() technique can get distinctive values from a listing by making a dictionary with the listing components as keys. Since dictionaries can’t have duplicate keys, this technique mechanically removes duplicates and returns a listing of distinctive values.
Instance
my_list = [1, 2, 3, 3, 4, 5, 5, 6]
unique_values = listing(dict.fromkeys(my_list))
print(unique_values)
Output
[1, 2, 3, 4, 5, 6]
Utilizing the Counter() Operate
The Counter() operate from the collections module is a robust instrument for counting the occurrences of components in a listing. We are able to acquire the distinctive values from the unique listing by changing the Counter object into a listing.
Instance
from collections import Counter
my_list = [1, 2, 3, 3, 4, 5, 5, 6]
unique_values = listing(Counter(my_list))
print(unique_values)
Output
[1, 2, 3, 4, 5, 6]
Utilizing the Pandas Library
The Pandas library supplies a complete set of information manipulation and evaluation instruments. It gives a novel() operate for acquiring distinctive values from a listing or a pandas Sequence object.
Instance
import pandas as pd
my_list = [1, 2, 3, 3, 4, 5, 5, 6]
unique_values = pd.Sequence(my_list).distinctive().tolist()
print(unique_values)
Output
[1, 2, 3, 4, 5, 6]
Additionally learn: 15 Important Python Record Features & Methods to Use Them (Up to date 2024)
Comparability of Strategies
Now, let’s examine the above strategies primarily based on their efficiency, reminiscence utilization, and dealing with of mutable and immutable components.
Efficiency
Relating to efficiency, the set() operate and listing comprehension technique are the quickest methods to acquire distinctive values from a listing. They’ve a time complexity of O(n), the place n is the size of the listing. The dict.fromkeys() technique and Counter() operate even have a time complexity of O(n), however they contain extra steps that make them barely slower. The Pandas library, whereas highly effective for knowledge evaluation, is relatively slower as a result of its overhead.
Reminiscence Utilization
By way of reminiscence utilization, the set() operate and listing comprehension technique are memory-efficient as they remove duplicates straight from the listing. The dict.fromkeys() technique and Counter() operate create extra knowledge buildings, which can eat extra reminiscence. As a complete instrument, the Pandas library requires extra reminiscence for its knowledge buildings and operations.
Dealing with Mutable and Immutable Parts
All of the strategies mentioned above work effectively with each mutable and immutable components. Whether or not the listing comprises integers, strings, tuples, or customized objects, these strategies can deal with them successfully and supply distinctive values accordingly.
You may as well learn: Python Record Applications For Absolute Rookies
Examples of Getting Distinctive Values from a Record in Python
Let’s discover a couple of extra examples to grasp easy methods to get distinctive values from a listing in numerous situations.
Instance 1: Getting Distinctive Values from a Record of Tuples
We are able to use listing comprehension if our listing comprises tuples and we need to acquire distinctive values primarily based on a selected aspect of every tuple.
my_list = [(1, 'a'), (2, 'b'), (3, 'a'), (4, 'c'), (5, 'b')]
unique_values = [x for i, x in enumerate(my_list) if x[1] not in [y[1] for y in my_list[:i]]]
print(unique_values)
Output
[(1, ‘a’), (2, ‘b’), (4, ‘c’)]
Instance 2: Discovering Distinctive Values in a Nested Record
If our listing is nested, and we need to acquire distinctive values throughout all ranges, we are able to use the itertools library to flatten the listing after which apply the specified technique.
import itertools
my_list = [[1, 2, 3], [2, 3, 4], [3, 4, 5]]
flattened_list = listing(itertools.chain.from_iterable(my_list))
unique_values = listing(set(flattened_list))
print(unique_values)
Output
[1, 2, 3, 4, 5]
Ideas and Methods for Effectively Getting Distinctive Values
Sorting the Record Earlier than Eradicating Duplicates
If the order of distinctive values isn’t necessary, sorting the listing earlier than eradicating duplicates can enhance efficiency. It is because sorting brings comparable components collectively, making figuring out and eradicating duplicates simpler.
Utilizing the setdefault() Methodology for Nested Lists
When working with nested lists, the setdefault() technique can be utilized to acquire distinctive values effectively. It permits us to create a dictionary with the weather as keys and their occurrences as values. We are able to acquire the distinctive values by changing the dictionary keys again into a listing.
Utilizing the itertools Library for Superior Operations
The itertools library supplies highly effective instruments for superior operations on lists, together with acquiring distinctive values. Features like chain(), groupby(), and combos() can be utilized to control and extract distinctive values from advanced knowledge buildings.
Conclusion
On this article, we explored varied strategies to get distinctive values from a listing in Python. We mentioned the significance of acquiring distinctive values and in contrast totally different strategies primarily based on their efficiency, reminiscence utilization, and dealing with of mutable and immutable components. We additionally offered examples and ideas for effectively getting distinctive values. By understanding these strategies and their purposes, you’ll be able to improve your Python programming expertise and enhance the effectivity of your code.